A pointer to struct in C is a variable that stores the address of a struct variable. This allows you to access the members of the struct variable indirectly, using the pointer variable.
To declare a pointer to struct, you use the struct
keyword followed by the name of the struct and the pointer variable name, with an asterisk (*) symbol. For example, the following code declares a pointer to a struct called Person
:
struct Person *person_ptr;
The person_ptr
variable now stores the address of a Person
struct variable. You can access the members of the struct variable using the pointer variable, using the arrow operator (->). For example, the following code prints the name of the person stored in the person_ptr
variable:
printf("%s\n", person_ptr->name);
Why use pointers to structs in C?
There are a few reasons why you might want to use pointers to structs in C:
- To pass structs to functions. When you pass a struct to a function, you are actually passing the address of the struct to the function. This can be useful if you need to modify the struct in the function.
- To create dynamic structs. You can use pointers to structs to create dynamic structs. This means that you can create a struct at runtime, without having to declare it in the source code.
- To improve performance. In some cases, pointers to structs can improve the performance of your code. This is because the compiler can optimize code that uses pointers to structs more easily than code that does not use pointers to structs.
Examples of pointers to structs in C
Here are some examples of how pointers to structs can be used in C programs:
- Passing structs to functions. As mentioned in the previous section, you can use pointers to structs to pass structs to functions. For example, the following code defines a function that takes a pointer to a struct as its argument:
Thevoid print_person(struct Person *person) { printf("%s\n", person->name); printf("%d\n", person->age); printf("%s\n", person->address); }
print_person()
function takes a pointer to aPerson
struct as its argument. The function then uses the pointer variable to access the members of the struct and prints them to the console.
- Creating dynamic structs. You can use pointers to structs to create dynamic structs. For example, the following code creates a dynamic struct at runtime:
Thestruct Person *person = malloc(sizeof(struct Person)); person->name = "John Doe"; person->age = 30; person->address = "123 Main Street";
malloc()
function allocates memory for aPerson
struct on the heap. Theperson
pointer variable now stores the address of the allocated struct. We can then assign values to the members of the struct.
- Improving performance. In some cases, pointers to structs can improve the performance of your code. This is because the compiler can optimize code that uses pointers to structs more easily than code that does not use pointers to structs. For example, the following code defines a function that calculates the distance between two points:
float distance(struct Point *p1, struct Point *p2) { float x = p1->x - p2->x; float y = p1->y - p2->y; return sqrt(x * x + y * y); }
The
distance()
function calculates the distance between two points. The function takes two pointers toPoint
structs as its arguments. The function then uses the pointers to access the x and y coordinates of the points and calculates the distance between them.The
distance()
function can be optimized by the compiler if it knows that thep1
andp2
pointers are not going to change. This is because the compiler can then calculate the distance between the points without having to dereference the pointers.
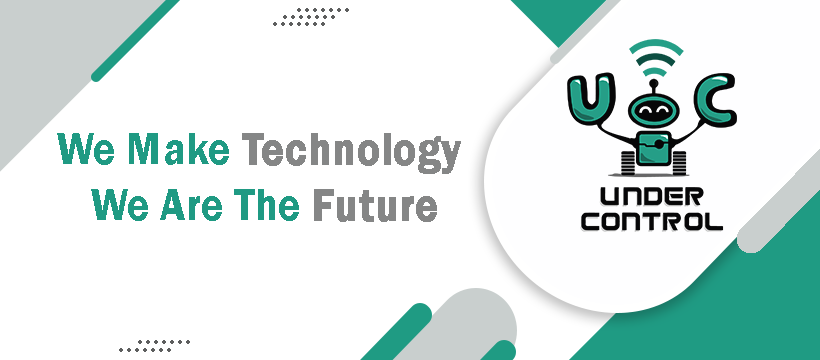