Introduction to C Operators
In C programming, operators are symbols that perform operations on variables, constants, and expressions. There are many different types of operators in C, each with its own purpose.
Types of C Operators
C operators can be classified into the following categories:
- Arithmetic operators: These operators are used to perform mathematical operations on numbers. Examples of arithmetic operators include
+
,-
,*
,/
,%
,++
, and--
.- Relational operators: These operators are used to compare two values and determine whether they are equal, greater than, less than, etc. Examples of relational operators include
==
,!=
,<
,>
,<=
, and>=
.- Logical operators: These operators are used to combine the results of two or more relational operators. Examples of logical operators include
&&
,||
, and!
.- Bitwise operators: These operators are used to perform operations on the bits of a binary number. Examples of bitwise operators include
&
,|
,^
,~
,<<
, and>>
.- Assignment operators: These operators are used to assign the value of an expression to a variable. Examples of assignment operators include
=
,*=
,/=
,%=
,+=
,-=
,<<=
, and>>=
.- Miscellaneous operators: These operators include the
sizeof
operator, the?:
operator, and the comma operator.
Operator Precedence
The order in which operators are evaluated is called operator precedence. Operators with higher precedence are evaluated before operators with lower precedence. For example, in the expression 1 + 2 * 3
, the multiplication operator (*
) is evaluated before the addition operator (+
), because multiplication has higher precedence than addition.
Operator Symbols
The symbols for C operators are as follows:
Operator Examples
Here are some examples of how C operators are used:
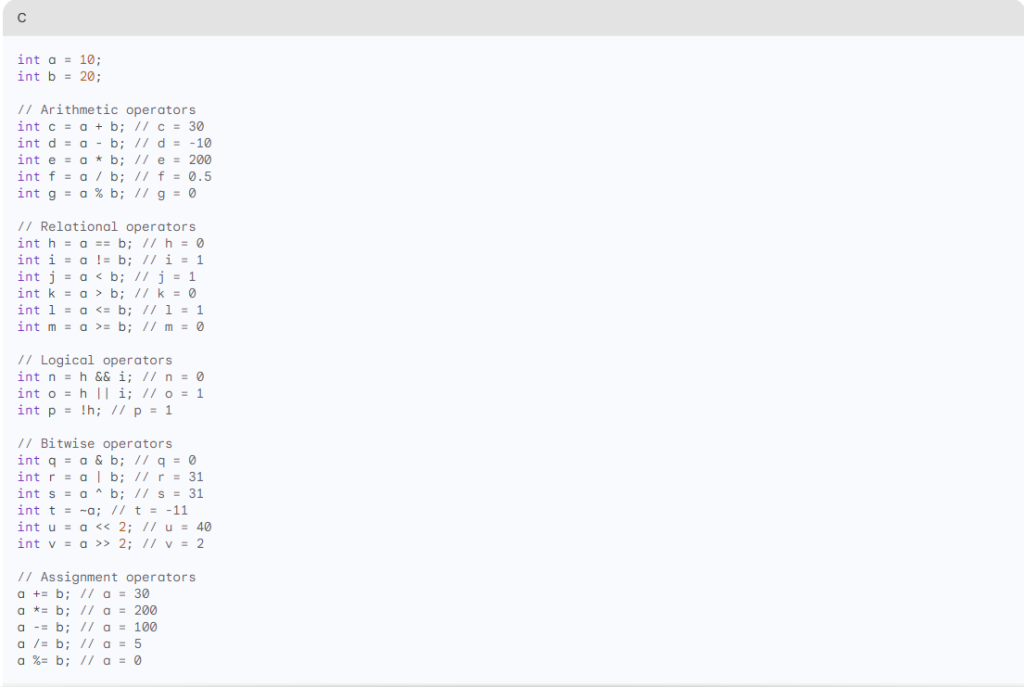
Conclusion
C operators are powerful tools that can be used to perform a variety of tasks. By understanding the different types of operators and their precedence, you can write more concise and efficient C code.