In C programming language, a dangling pointer is a pointer that points to a memory location that has been deallocated. This can happen when a variable goes out of scope, or when the memory is explicitly freed using the free()
function.
Dangling pointers are dangerous because they can lead to undefined behavior. If you try to dereference a dangling pointer, the program may crash, or it may read or write data from an invalid memory location.
How do dangling pointers occur?
Dangling pointers can occur in a few different ways:
- When a variable goes out of scope: When a variable goes out of scope, its memory is automatically deallocated. However, if the variable is pointing to another variable, the pointer will still point to the memory location of the deallocated variable. This is a dangling pointer.
- When the memory is explicitly freed using the
free()
function: Thefree()
function is used to deallocate memory that has been dynamically allocated using themalloc()
function. However, if the pointer is not updated to NULL after the memory is freed, it will still point to the deallocated memory location. This is a dangling pointer.- When a function returns a pointer to a local variable: When a function returns a pointer to a local variable, the pointer will still point to the memory location of the local variable after the function returns. This is a dangling pointer if the local variable goes out of scope after the function returns.
How to avoid dangling pointers
There are a few ways to avoid dangling pointers in C programming language:
- Always initialize pointers to NULL before you use them. This will prevent you from accidentally dereferencing a pointer that hasn’t been initialized.
- Use the
free()
function correctly. When you free memory, make sure to update the pointer to NULL so that you don’t accidentally dereference it later.- Don’t return pointers to local variables from functions. If you need to return a pointer from a function, make sure it is a pointer to a global variable or a dynamically allocated variable.
- Use the
valgrind
tool to check your code for memory errors.valgrind
is a memory debugging tool that can help you to find and fix dangling pointers in your code.
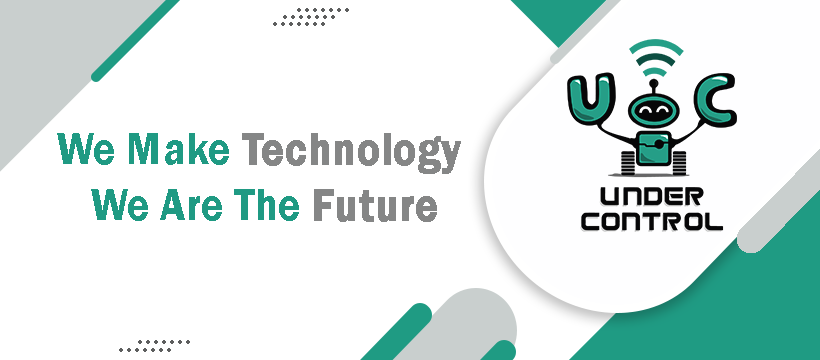