If Statements in C
If statements are one of the most basic and important control flow statements in the C programming language. They allow you to execute code only if a certain condition is met.
The syntax for an if statement in C is as follows:
if (condition) {
// code to be executed if condition is true
}
The condition
can be any expression that evaluates to a Boolean value, such as true
or false
. If the condition is true, the code inside the braces {}
will be executed. If the condition is false, the code inside the braces will be skipped.
You can also use an else statement with an if statement to execute different code depending on whether the condition is true or false. The syntax for an else statement is as follows:
if (condition) {
// code to be executed if condition is true
} else {
// code to be executed if condition is false
}
The else statement will only be executed if the condition in the if statement is false.
Here is an example of an if statement in C:
int age = 18;
if (age >= 18) {
printf("You are an adult.\n");
}
In this example, the age
variable is assigned the value of 18. The if statement checks if the value of age
is greater than or equal to 18. If it is, the code inside the braces {}
will be executed, which in this case is the printf()
function. The printf()
function will print the text “You are an adult.” to the console.
Here is another example of an if statement with an else statement in C:
int number = 5;
if (number % 2 == 0) {
printf("The number is even.\n");
} else {
printf("The number is odd.\n");
}
In this example, the number
variable is assigned the value of 5. The if statement checks if the remainder of number
divided by 2 is equal to 0. If it is, the code inside the braces {}
will be executed, which in this case is the printf()
function. The printf()
function will print the text “The number is even.” to the console. If the remainder of number
divided by 2 is not equal to 0, the code inside the else statement will be executed, which in this case is the printf()
function. The printf()
function will print the text “The number is odd.” to the console.
If statements are a powerful tool that can be used to control the flow of your code. They can be used to make your code more efficient and easier to read.
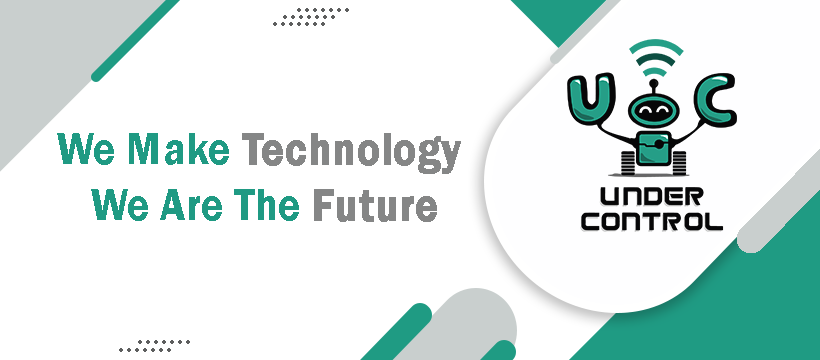