Pointers are one of the most powerful features of the C programming language. They allow us to manipulate memory directly, which can be very useful in many programming scenarios.
There are a number of operations that can be performed on pointers in C. Some of the most common operations include:
- Declaring a pointer: To declare a pointer, we use the
*
operator. For example, the following code declares a pointer namedp
that can store the address of an integer:
int* p;
- Initializing a pointer: To initialize a pointer, we can use the
&
operator to get the address of another variable. For example, the following code initializes the pointerp
to the address of the variablen
:
int n = 10;
int* p = &n;
- Dereferencing a pointer: To dereference a pointer, we use the
*
operator. This means that we are accessing the value of the variable that the pointer is pointing to. For example, the following code prints the value of the variablen
by dereferencing the pointerp
:
printf("The value of n is %d.\n", *p);
- Incrementing/decrementing a pointer: We can increment or decrement a pointer by using the
++
or--
operators. This will move the pointer to the next or previous memory location, respectively. For example, the following code will increment the pointerp
by one:
p++;
- Adding/subtracting a constant to/from a pointer: We can add or subtract a constant to or from a pointer by using the
+
or-
operators. This will move the pointer to the memory location that is the specified constant number of memory locations away. For example, the following code will move the pointerp
to the memory location that is 10 memory locations away:
p = p + 10;
- Subtracting two pointers: We can subtract two pointers to get the difference between the memory addresses that they are pointing to. This difference is the number of memory locations between the two pointers. For example, the following code will subtract the pointer
p
from the pointerq
and store the difference in the variablen
:
int n = q - p;
- Comparing two pointers: We can compare two pointers to see if they are equal or not. We can use the
==
,!=
,<
,>
,<=
, and>=
operators to compare pointers. For example, the following code will compare the pointersp
andq
and print “The pointers are equal” if they are equal and “The pointers are not equal” if they are not equal:
if (p == q) {
printf("The pointers are equal.\n");
} else {
printf("The pointers are not equal.\n");
}
These are just some of the most common operations that can be performed on pointers in C. Pointers can be a powerful tool, but it is important to use them carefully. It is important to understand how pointers work before using them in your code.
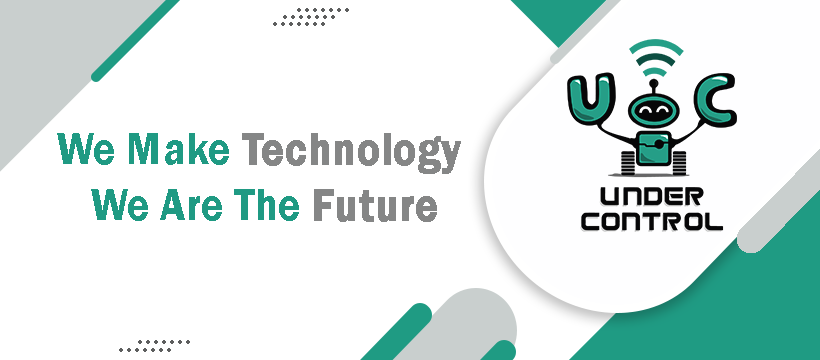