A struct in C is a user-defined data type that can be used to group items of possibly different types into a single type. The struct keyword is used to define the structure in the C programming language. The items in the structure are called its members and they can be of any valid data type.
For example, the following code defines a struct called Person
that has three members: name
, age
, and address
:
struct Person {
char *name;
int age;
char *address;
};
Once a struct is defined, we can create variables of that type. For example, the following code creates two Person
variables:
struct Person person1;
struct Person person2;
The person1
and person2
variables can now be used to store data about two different people. For example, we could assign the following values to the person1
variable:
C
person1.name = "John Doe";
person1.age = 30;
person1.address = "123 Main Street";
We can then access the data in the person1
variable using the dot notation. For example, the following code prints the name of the person stored in the person1
variable:
printf("%s\n", person1.name);
Why use structs in C?
Structs are a powerful tool that can be used to improve the readability, maintainability, and efficiency of C programs. Here are some of the reasons why you might want to use structs in your C programs:
- To group related data together. Structs can be used to group related data together into a single unit. This can make your code more readable and maintainable, as you only have to worry about one variable for each group of data.
- To save memory. Structs can be used to save memory by storing multiple data types in a single variable. This can be especially useful if you are working with a large amount of data.
- To improve efficiency. Structs can be used to improve efficiency by allowing you to access multiple data types with a single statement. This can be especially useful if you are working with a large amount of data.
Examples of structs in C
Here are some examples of how structs can be used in C programs:
- Storing information about a person. As mentioned in the previous section, structs can be used to store information about a person. For example, we could use a struct to store the person’s name, age, address, and phone number.
- Storing the game state in a game. In a game, structs can be used to store the game state. For example, we could use a struct to store the player’s health, score, and position on the map.
- Storing the configuration of a device. In a device driver, structs can be used to store the configuration of the device. For example, we could use a struct to store the device’s address, IRQ, and memory map.
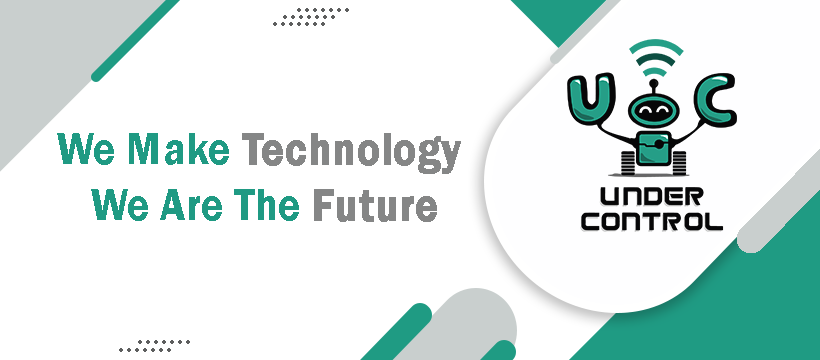