An array in C is a data structure that can store multiple values of the same data type. Arrays are declared using the following syntax:
dataType arrayName[arraySize];
Where dataType
is the data type of the elements in the array, arrayName
is the name of the array, and arraySize
is the number of elements in the array.
For example, the following code declares an array of 10 integers:
int numbers[10];
Once an array is declared, you can initialize it with values using the following syntax:
arrayName[index] = value;
Where index
is the index of the element in the array, and value
is the value to be stored in the element.
For example, the following code initializes the first 5 elements of the numbers
array with the values 1, 2, 3, 4, and 5:
numbers[0] = 1;
numbers[1] = 2;
numbers[2] = 3;
numbers[3] = 4;
numbers[4] = 5;
You can access the elements of an array using the following syntax:
arrayName[index];
Where index
is the index of the element you want to access.
For example, the following code prints the value of the first element of the numbers
array:
printf("%d\n", numbers[0]);
Arrays can be used to store a variety of data, such as numbers, strings, and characters. They are a powerful tool for storing and accessing data in C programming.
Advantages of Arrays in C
There are several advantages to using arrays in C programming. Some of the most important advantages include:
- Efficiency: Arrays are a very efficient way to store and access data. The elements of an array are stored in contiguous memory locations, which makes it very fast to access them.
- Simplicity: Arrays are relatively simple to use. The syntax for declaring and accessing arrays is straightforward.
- Flexibility: Arrays can be used to store a variety of data types. This makes them a versatile tool for storing data in C programming.
Disadvantages of Arrays in C
There are also a few disadvantages to using arrays in C programming. Some of the most important disadvantages include:
- Fixed size: Arrays have a fixed size. This means that you cannot add or remove elements from an array after it has been declared.
- Limited data types: Arrays can only store data of a single data type. This can be a limitation if you need to store data of multiple data types.
- Danger of out of bounds errors: It is possible to access elements of an array that are outside of the array’s bounds. This can cause a segmentation fault, which will crash your program.
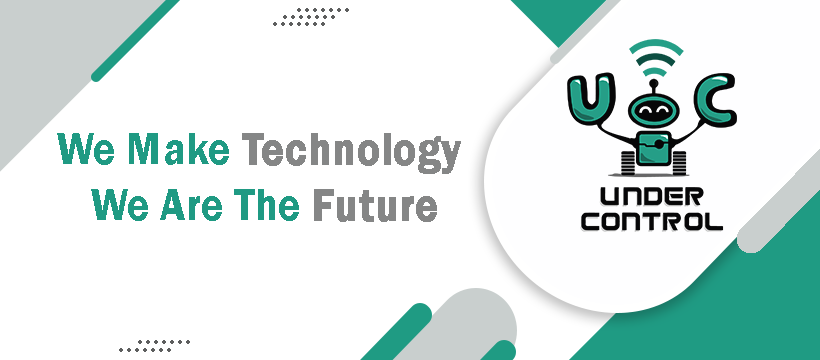