Bubble sort is a simple sorting algorithm that works by repeatedly comparing adjacent elements in an array and swapping them if they are in the wrong order. The algorithm continues until no more swaps are necessary, at which point the array is sorted.
Here is the pseudocode for bubble sort:
procedure bubble_sort(array)
for i from 0 to n - 1
for j from 0 to n - i - 1
if array[j] > array[j + 1]
swap(array[j], array[j + 1])
Here is the C code for bubble sort:
void bubble_sort(int array[], int size) {
for (int i = 0; i < size - 1; i++) {
for (int j = 0; j < size - i - 1; j++) {
if (array[j] > array[j + 1]) {
int temp = array[j];
array[j] = array[j + 1];
array[j + 1] = temp;
}
}
}
}
To use the bubble sort algorithm, you would first need to create an array of integers. Then, you would call the bubble_sort function, passing in the array and the size of the array as parameters. The function will sort the array in ascending order.
Here is an example of how to use the bubble sort algorithm in C:
int main() {
int array[] = {10, 5, 2, 7, 1, 8};
int size = sizeof(array) / sizeof(array[0]);
bubble_sort(array, size);
for (int i = 0; i < size; i++) {
printf("%d ", array[i]);
}
return 0;
}
This code will print the following output:
1 2 5 7 8 10
As you can see, the bubble sort algorithm has sorted the array in ascending order.
Bubble sort is a simple sorting algorithm, but it is not very efficient. It has a worst-case time complexity of O(n^2), where n is the size of the array. This means that the time it takes to sort the array grows quadratically with the size of the array.
There are more efficient sorting algorithms available, such as quicksort and merge sort. However, bubble sort is a good algorithm to learn for beginners because it is easy to understand and implement.
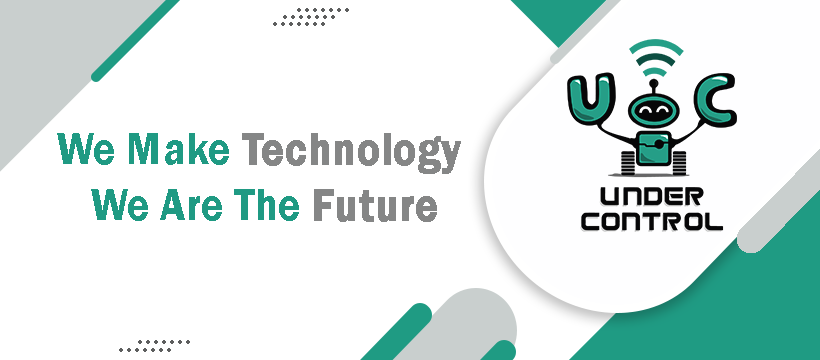