A do while loop is a repetition control structure that allows programmers to write a loop that will be executed at least once, even if the condition is false at the beginning. The do while loop has two parts:
- Body of the loop: This is the block of statements that is executed repeatedly as long as the condition statement is true.
- Condition statement: This statement is evaluated after each iteration of the loop. If the condition is true, the body of the loop is executed again. If the condition is false, the loop terminates.
The general syntax of a do while loop in C is as follows:
do {
// Body of the loop
} while (condition statement);
Here is an example of a do while loop that prints the numbers from 1 to 10:
int i = 1;
do {
printf("%d\n", i);
i++;
} while (i <= 10);
This do while loop first executes the body of the loop, which is the printf()
statement. The printf()
statement prints the value of i
to the console. After the body of the loop is executed, the condition statement is evaluated, which is i <= 10
. If the condition is true, the body of the loop is executed again. If the condition is false, the loop terminates.
Do while loops are a useful tool in C programming. They can be used to implement loops that must be executed at least once, even if the condition is false at the beginning. They can also be used to implement loops that need to be executed a specific number of times, but the number of iterations is not known in advance.
Here are some additional things to keep in mind about do while loops in C:
- The body of the loop is always executed at least once, even if the condition statement is false at the beginning.
- The condition statement is evaluated after the body of the loop is executed.
- The body of the loop can be a single statement or a block of statements.
Here is a table comparing the do while loop to the while loop:
Feature | Do While Loop | While Loop |
Execution of body of loop | Always executed at least once | May not be executed at all |
Evaluation of condition statement | After body of loop is executed | Before body of loop is executed |
Use cases | When you need to execute the loop at least once, even if the condition is false at the beginning. | When you need to execute the loop as long as the condition is true. |
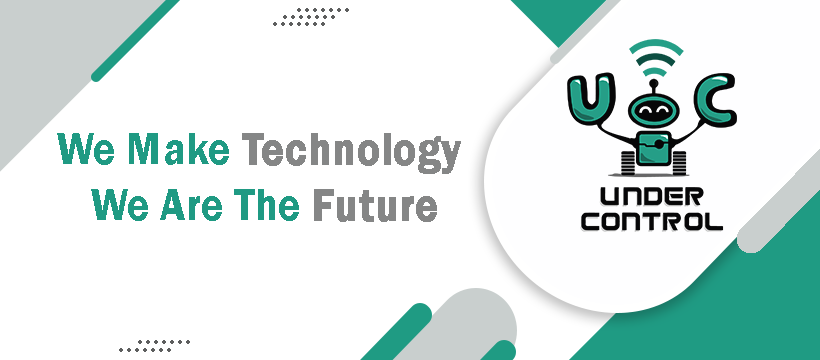