An enum, or enumeration, is a user-defined data type in C that consists of a set of named constants. Enums are often used to represent sets of values that have a natural ordering, such as the days of the week, the months of the year, or the possible states of a machine.
To define an enum in C, you use the enum
keyword. For example, the following code defines an enum called day
that represents the days of the week:
enum day {
monday,
tuesday,
wednesday,
thursday,
friday,
saturday,
sunday
};
The enum day
enum has seven members: monday, tuesday, wednesday, thursday, friday, saturday, and sunday. The members of an enum are automatically assigned consecutive integers starting from 0. So, the member monday
has the value 0, the member tuesday
has the value 1, and so on.
You can use enums in your C code just like any other data type. For example, the following code declares a variable called day_of_week
of type enum day
:
enum day day_of_week;
You can then set the value of day_of_week
to one of the members of the enum day
enum. For example, the following code sets the value of day_of_week
to monday
:
day_of_week = monday;
You can also use enums in switch statements. For example, the following code uses a switch statement to print the day of the week:
switch (day_of_week) {
case monday:
printf("It's Monday!");
break;
case tuesday:
printf("It's Tuesday!");
break;
case wednesday:
printf("It's Wednesday!");
break;
case thursday:
printf("It's Thursday!");
break;
case friday:
printf("It's Friday!");
break;
case saturday:
printf("It's Saturday!");
break;
case sunday:
printf("It's Sunday!");
break;
}
Enums are a powerful tool that can be used to make your C code more readable and maintainable. They are especially useful for representing sets of values that have a natural ordering.
Here are some additional things to keep in mind about enums in C:
- The members of an enum must be unique. You cannot have two members with the same name.
- The members of an enum can be of any data type. However, it is usually best to use an integer data type for the members of an enum.
- You can use the
typedef
keyword to create a typedef for an enum. This can make your code more readable and maintainable.
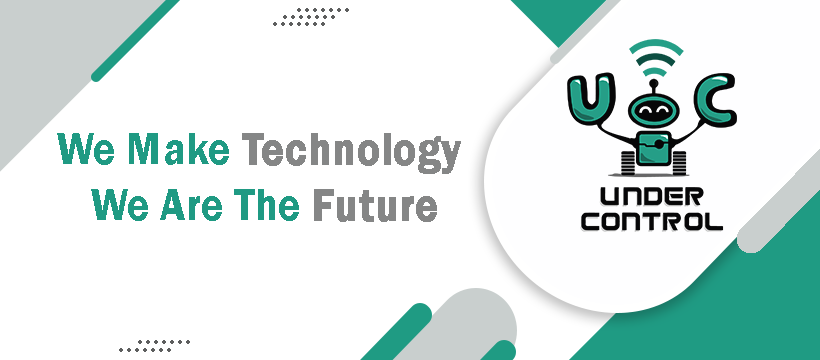