A for loop is a repetition control structure that allows programmers to write a loop that will be executed a specific number of times. The for loop has three parts:
- Initialization statement: This statement is executed only once, before the loop starts. It is used to initialize the loop variable.
- Condition statement: This statement is evaluated before each iteration of the loop. If the condition is true, the body of the loop is executed. If the condition is false, the loop terminates.
- Update statement: This statement is executed after each iteration of the loop. It is used to update the loop variable.
The general syntax of a for loop in C is as follows:
for (initialization statement; condition statement; update statement) {
// Body of the loop
}
Here is an example of a for loop that prints the numbers from 1 to 10:
for (int i = 1; i <= 10; i++) {
printf("%d\n", i);
}
This for loop first initializes the loop variable i
to 1. Then, it evaluates the condition statement, which is i <= 10
. If the condition is true, the body of the loop is executed. The body of the loop in this case is the printf()
statement, which prints the value of i
to the console. After the body of the loop is executed, the update statement is executed, which increments the value of i
by 1. This process repeats until the condition statement is false, which is when i
is equal to 11.
For loops are a very versatile tool in C programming. They can be used to iterate over arrays, lists, and other data structures. They can also be used to perform repetitive tasks, such as printing a table of values or calculating a mathematical series.
Here are some additional things to keep in mind about for loops in C:
- The initialization statement, condition statement, and update statement can be empty, but at least one of them must be present.
- The semicolons after the initialization statement, condition statement, and update statement are required.
- The body of the loop can be a single statement or a block of statements.
- The update statement can be used to change the value of the loop variable in any way, as long as it is legal C code.
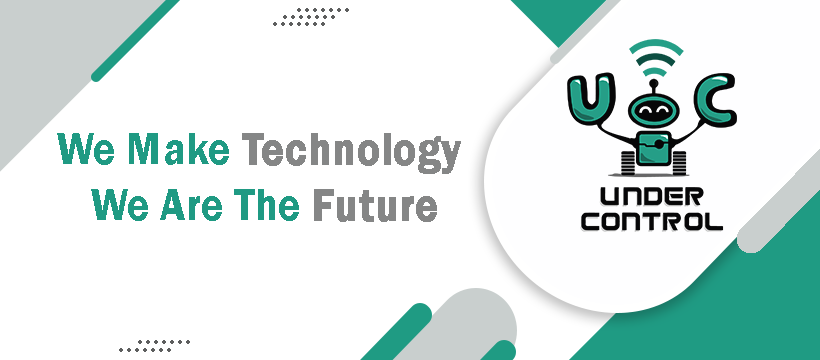