The preprocessor is a program that is run before the compiler compiles your C code. It reads your source code and performs a number of tasks, such as:
- Replacing macros with their defined values
- Including header files
- Conditional compilation
- Line control
Preprocessor directives are instructions that tell the preprocessor what to do. They start with the #
symbol. Here are some of the most common preprocessor directives:
- #define defines a macro. A macro is a name that is replaced with a value when the preprocessor processes your code. For example, you could define a macro called
MAX_SIZE
to be 100. Then, you could use the macro in your code like this:int array[MAX_SIZE];
The preprocessor would replace MAX_SIZE
with the value 100, so the above line of code would create an array with 100 elements.
- #include includes a header file. A header file is a file that contains declarations for functions, variables, and macros. When you use the
#include
directive, the preprocessor will copy the contents of the header file into your source code. This is useful for avoiding duplicate declarations and for making your code more modular.- #if, #ifdef, and #ifndef are used for conditional compilation. These directives allow you to specify conditions that must be met before the preprocessor will process certain parts of your code. For example, you could use
#if
to only compile a section of code if a certain macro is defined.- #else and #endif are used to terminate conditional compilation blocks. The
#else
directive specifies the code that will be executed if the condition in the#if
directive is not met. The#endif
directive marks the end of a conditional compilation block.- #line controls the line number that is used for error messages. This can be useful for debugging your code.
Preprocessor directives can be a powerful tool for improving the readability and maintainability of your C code. By using preprocessor directives, you can make your code more concise, reusable, and portable.
Here are some additional resources that you may find helpful:
- C Preprocessor Tutorial: https://www.tutorialspoint.com/cprogramming/c_preprocessors.htm
- Preprocessor Directives in C: https://www.geeksforgeeks.org/cc-preprocessors/
- The C Preprocessor: https://en.wikipedia.org/wiki/C_preprocessor
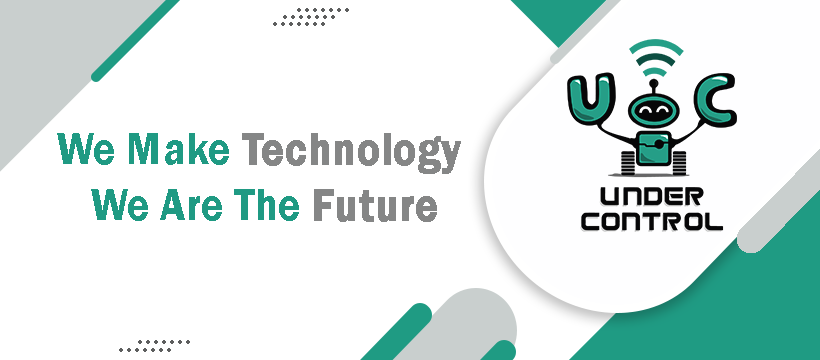