The switch statement in C is a multiway branch statement. It allows a value to change control of execution. They are a substitute for long if statements that compare a variable to several integral values. The switch statement is a more efficient way to write code that performs different actions based on different conditions.
The syntax for a switch statement in C is as follows:
switch(expression) {
case value1:
// statements to be executed when expression is equal to value1
break;
case value2:
// statements to be executed when expression is equal to value2
break;
...
default:
// statements to be executed when expression is not equal to any of the cases
}
The expression in the switch statement is evaluated once. The value of the expression is then compared to the values of each case label. If there is a match, the statements following the matching label are executed until a break statement is reached. If there is no match, the default statements are executed.
The break statement is used to terminate the switch statement and continue execution with the next statement. If the break statement is not used, all the statements after the matching case label will be executed. This is known as “fall through”.
The default statements are executed if the value of the expression does not match any of the case labels. The default statements are optional. If they are not present, and the value of the expression does not match any of the case labels, the switch statement will simply terminate.
Here is an example of a switch statement in C:
int main() {
int day = 3;
switch(day) {
case 1:
printf("Monday\n");
break;
case 2:
printf("Tuesday\n");
break;
case 3:
printf("Wednesday\n");
break;
case 4:
printf("Thursday\n");
break;
case 5:
printf("Friday\n");
break;
case 6:
printf("Saturday\n");
break;
case 7:
printf("Sunday\n");
break;
default:
printf("Invalid day\n");
}
return 0;
}
This program will print the day of the week corresponding to the value of the variable day
. For example, if the value of day
is 3, the program will print “Wednesday”.
The switch statement is a powerful tool that can be used to write efficient and readable code. It is a good choice for situations where you need to perform different actions based on the value of a variable.
Here are some additional tips for using the switch statement in C:
- Use the switch statement when you have a variable that can have a limited number of possible values.
- Use the break statement to terminate the switch statement and continue execution with the next statement.
- Use the default statements to handle cases where the value of the expression does not match any of the case labels.
- Avoid using the switch statement for complex logic. In these cases, it is better to use an if-else statement.
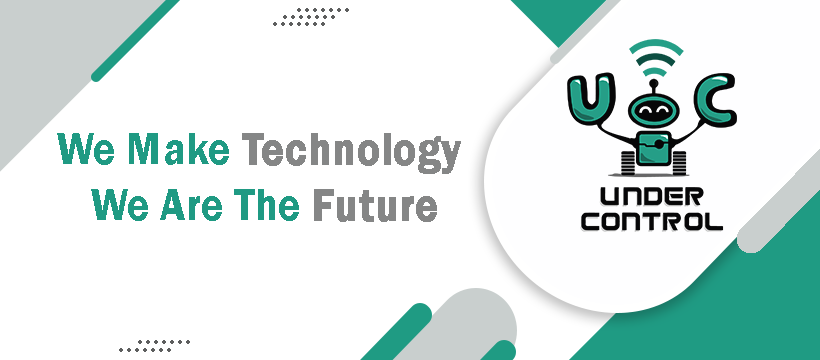