A while loop is a repetition control structure that allows programmers to write a loop that will be executed as long as a given condition is true. The while loop has two parts:
- Condition statement: This statement is evaluated before each iteration of the loop. If the condition is true, the body of the loop is executed. If the condition is false, the loop terminates.
- Body of the loop: This is the block of statements that is executed repeatedly as long as the condition statement is true.
The general syntax of a while loop in C is as follows:
while (condition statement) {
// Body of the loop
}
Here is an example of a while loop that prints the numbers from 1 to 10:
int i = 1;
while (i <= 10) {
printf("%d\n", i);
i++;
}
This while loop first initializes the loop variable i
to 1. Then, it evaluates the condition statement, which is i <= 10
. If the condition is true, the body of the loop is executed. The body of the loop in this case is the printf()
statement, which prints the value of i
to the console. After the body of the loop is executed, the loop variable i
is incremented by 1. This process repeats until the condition statement is false, which is when i
is equal to 11.
While loops are a very versatile tool in C programming. They can be used to iterate over arrays, lists, and other data structures. They can also be used to perform repetitive tasks, such as printing a table of values or calculating a mathematical series.
Here are some additional things to keep in mind about while loops in C:
- The condition statement must be a boolean expression.
- The body of the loop can be a single statement or a block of statements.
- If the condition statement is always true, the loop will never terminate.
- If the condition statement is always false, the loop will never be executed.
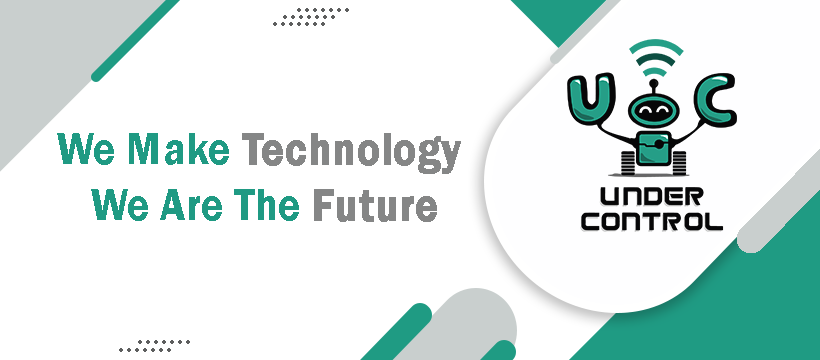